Python 入門 5 | 函式、模組與套件
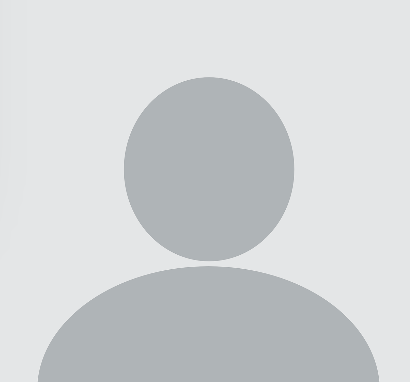
劉晉嘉
資工系二年級
- GDG on Campus NTPU 核心幹部 技術組成員
- 擅長撰寫 C++
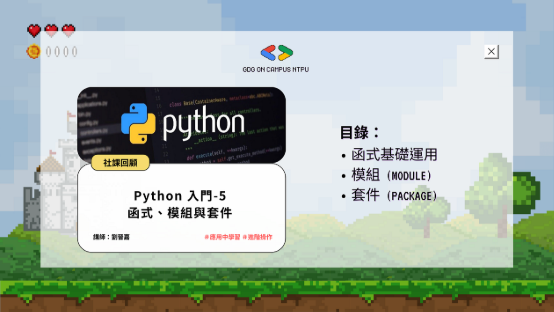
講座內容摘要
【社課回顧】Python 入門-5 | 函式、模組與套件
一、函式基礎運用
1. 定義函式:使用 def 關鍵字定義函式。
def greet(name):
` `return f"Hello, {name}!" # 使用 f-string 格式化字串,將 name 值回傳進函式
2. 調用函式:通過函式名和參數調用函式。
# 承上
print(greet("Alice")) # 輸出: Hello, Alice!
3. 默認參數值:可以為參數設置默認值。
def greet(name="World"):
` `return f"Hello, {name}!"
print(greet()) # 輸出: Hello, World!
print(greet("Bob")) # 輸出: Hello, Bob!
4. 返回值:使用 return 關鍵字,可讓函式回傳一個值。
def add(a, b):
` `return a + b
result = add(3, 5)
print(result) # 輸出: 8
5. 應用練習:寫一個函式判斷是不是質數。
# 參考解答
def isPrime(n):
` `for i in range(2, int(n\*\*0.5) + 1):
` `if n % i == 0:
` `return False
` `return True
print(isPrime(13)) # 輸出: True
print(isPrime(15)) # 輸出: False
二、模組
1. 模組介紹:「模組」(Module)指的是一個包含 Python 代碼的文件,可以被其他 Python 程式導入和使用。模組讓我們可以把代碼拆分成更小、更易管理的部分,提高代碼的可讀性和重用性。
2. Python 兩大類型模組
- 內建模組:Python 自帶的模組,比如 math、os 等,這些模組可以直接導入使用。
- 自定義模組:由開發者自己編寫的模組,通常是包含在一個 .py 文件中的代碼。
3. 導入和使用內建模組
- 導入模組:使用 import 導入後,可以透過模組名調用其函式和變量。
import math # 導入內建的數學模組
# 使用 math 模組中的 sqrt() 函式來計算平方根
result = math.sqrt(16)
print(result) # 輸出: 4.0
- 導入模組中的特定功能:有時我們只需要模組中的某個函式或變量,這時可以使用 from ... import ... 語句來導入特定的部分。
from math import pi # 只導入 math 模組中的 pi 常數
print(pi) # 輸出: 3.141592653589793
- 使用別名:如果模組名稱太長或者與現有的變量名衝突,可以使用 as 為模組或函式設置別名。
import math as m # 將 math 模組重命名為 m
print(m.sqrt(25)) # 輸出: 5.0
4. 自定義模組
- 創建:將一些功能寫入一個 .py 文件中,即可將其視為一個模組。然後可以在其他文件中導入這個模組。
# mymodule.py 文件
def greet(name):
` `return f"Hello, {name}!"
- 導入:使用 import 導入自定義模組後,可以使用模組中的函式或變量。
# 在其他文件中使用自定義模組
import mymodule
message = mymodule.greet("Alice")
print(message) # 輸出: Hello, Alice!
三、套件
**1. 套件介紹:**當我們將程式碼模組化後,專案中的模組就會越來越多,這時就可以組織一個有如大資料夾的「套件」(Package),存入所有相似的模組。 *補充說明:原本套件的檔案目錄中須含有 __init__.py 檔案(初始化套件的程式碼),才會被 Pyhon 當成套件,但自 Python 3.3 起,這點已經不是必要的了,不過如果需要自訂初始化邏輯(如使用 __all__ 定義「可匯出成員」有哪些),仍然建議建立 __init__.py 檔案。
2. 導入和使用套件
- *:使用 * 符號導入整個套件中的所有模組。
# 假設有以下目錄結構:
# my\_package/
# \_\_init\_\_.py
# module1.py
# module2.py
# module3.py
# 注意:\_\_init\_\_.py 檔案中須明確定義
# e.g. \_\_all\_\_ = ["module1", "module2", "module3"]
from my\_package import \*
# 一次導入 module1.py、module2.py、module3.py
# 相當於 from my\_package import module1, module2, module3
- from-import(絕對導入):使用 from import 導入特定模組。
# 承上
# 假設 module1.py 定義了一個函式如下:
# def greet():
# print("Hello from module1!")
from my\_package import module1 # 也相當於直接 import module1
from my\_package import module2 # 也相當於直接 import module2
module1.greet() # 呼叫 module1 中的 greet 函式
- 套件內引用(相對導入):使用 from . 導入同層套件中的子模組。
# 承上
# 若此時,module2.py 也想要使用 module1 中的 greet 函式,
# 則我們可以在 module2.py 中撰寫:
from . import module1 # 導入與 module2.py 同層(都在 my\_package/下)的 module1
def call\_greet():
` `module1.greet() # 呼叫 module1 中的 greet 函式
辛苦啦~ 恭喜你完成了第五個,也是 Python 入門系列的最後一個【函式、模組與套件】學習單元!